As you may know, the frontend often needs to get information to show, to update, to persist, etc. This is usually done through a layer called API that is the information provider. In order to interact with this layer, there are different protocols such as:
- SOAP
- REST
- GraphQL
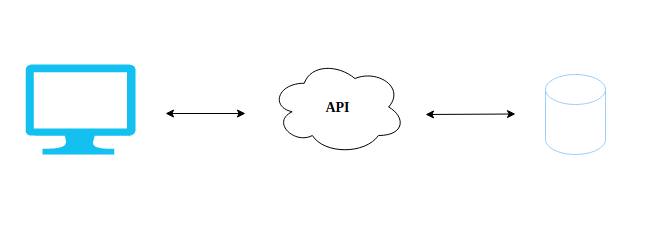
In this article, we’ll focus on GraphQL from the client-side and also show a brief example.
Basics / Why GraphQL? What’s QL?
GraphQL is a new protocol to interact with the backend, created by Facebook. QL stands for Query Language and this is because we’ll have the ability to request just the attributes that we require, nothing else.
Problem solved
Let’s say we need to show some information into App A and similar information into App B. Backend team will have to build two different web services, one per App, or if it’s the same web service, both Apps will receive additional and unwanted information.
One solution to solve this issue is to use a GraphQL API that will allow App A and App B to specify accurately the data each one wants.
Advantages
- GraphQL provides one source of information.
- From the client, you’ll be creating a kind of documentation in the query, because you will describe the data you want to receive.
- You can add a GraphQL layer to an existing REST API.
Examples
Let’s see an example of a request and response:
Request:
{
orders {
id
productsList {
product {
name
price
}
quantity
}
totalAmount
}
}
Response:
{
"data":{
"orders":[
{
"id":1,
"productsList":[
{
"product":{
"name":"orange",
"price":1.5
},
"quantity":100
}
],
"totalAmount":150
}
]
}
}
We can also do multiple requests in the same API call:
Request:
{
books {
id
name
category {
id
name
}
totalAmount
}
authors {
id
name
}
}
Response:
{
"data":{
"books":[
{
"id":1,
"name":"Rich dad poor dad",
"category":[
{
"id":1,
"name":"drama"
}
]
}
],
"authors":[
{
"id":1,
"name":"Robert Kiyosaki"
}
]
}
}
As you can see, in the request, we are creating a query where we specify what we want to get.
An important detail to mention is that this is a POST request and not a GET.
GraphQL vs Rest?
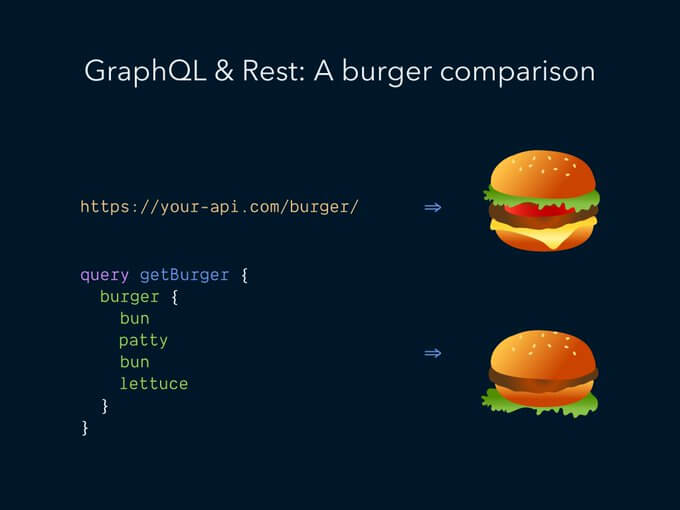
REST
- Multiple endpoints
- The data structure is controlled by the backend
- Provides structured access to resources
- The frontend has no control over how and what the data looks like
- The backend requires creating new APIs or adjusting existing ones to serve the correct amount and type of data. This can lead to a longer time for production changes and slow down the development process.
GraphQL
- Single endpoints that receive dynamic parameters
- Ready for multiple apps once implemented
- Just a single request in the body that includes a query that contains all the data requirements. This brings data into the frontend and reduces the amount of workload required on the backend to adjust to the changing needs of data requirements.
- The root field will return a data field with all the data you want and in the format you need it
- Allows for quick iteration and faster feedback for the business
Caching
In order to have better performance and avoid unnecessary requests to the backend, Graph QL works with a caching system.
In the following image, you can see how Apollo Client first looks for the data in the cache. If the data is not there, it sends a query to the server and caches the data.
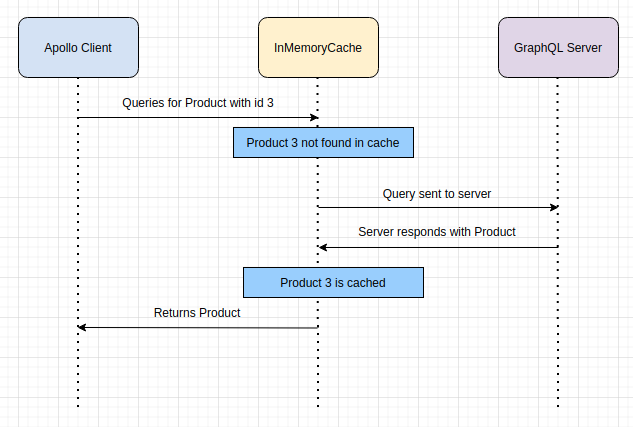
Now the data is cached, so when Apollo Client looks for it in the cache, it finds it and doesn’t send a query to the server.
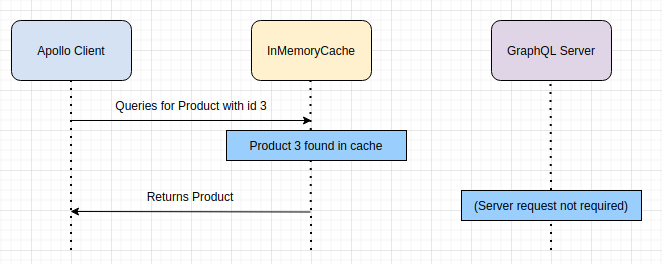
First steps / Installation
First we need to add some dependencies to our project:
npm install @apollo/client graphql
Then, we configure the ApolloProvider, the cache, and the ApolloClient to our project:
import React from "react";
import { Main } from "./views/Main";
import { ApolloProvider, InMemoryCache, ApolloClient } from "@apollo/client";
const client = new ApolloClient({
uri: "https://countries.trevorblades.com/",
cache: new InMemoryCache(),
});
function App() {
return (
<ApolloProvider client={client}>
<Main />
</ApolloProvider>
);
}
export default App;
A real example
After that, we just need to write our own query. In this case we are fetching Languages by code using a filter and using a parameter:
import React, { useState } from "react";
import { gql, useQuery } from "@apollo/client";
const GET_LANGUAGE_BY_CODE = gql`
query GetLanguageByCode($code: ID!) {
language(code: $code) {
name
}
}
`;
const GET_LANGUAGES_BY_CODE_FILTER = gql`
query GetLanguageByCode($code: String!) {
languages(filter: { code: { eq: $code } }) {
name
}
}
`;
const RenderLanguageByCode = ({ code }) => {
const { loading, error, data } = useQuery(GET_LANGUAGE_BY_CODE, {
variables: { code },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :{error}</p>;
return (
<div>
<p>{JSON.stringify(data?.language.name)}</p>
</div>
);
};
const RenderLanguagesByCodeFilter = ({ code }) => {
const { loading, error, data } = useQuery(GET_LANGUAGES_BY_CODE_FILTER, {
variables: { code },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :{error}</p>;
return (
<div>
<p>{JSON.stringify(data)}</p>
</div>
);
};
export const Home = () => {
const [code] = useState("af");
return (
<>
<RenderLanguageByCode code={code} />
<RenderLanguagesByCodeFilter code={code} />
</>
);
};
GraphQL and React (ApolloClient)
There are multiple libraries that allow us to interact with a GraphQL API from React, let’s mention the most popular ones:
- Apollo Client (most popular)
- Relay
- URQL
- React Query
Conclusions
GraphQL is a new approach that we may consider when we are deciding which kind of API we’ll use. I don’t say it’s better or worse than REST, but it’s another option that, depending on the case, could be better to use or not.
Personal advice: give it a try.